반응형
<html>
<head>
<meta charset="utf-8" />
<title>숫자야구</title>
</head>
<body>
<form id="form">
<input type="text" id="input" />
<button>확인</button>
</form>
<div id="logs"></div>
<script>
const $input = document.querySelector('#input');
const $form = document.querySelector('#form');
const $logs = document.querySelector('#logs');
// 랜덤 숫자 뽑기 (맞춰야 할 문제)
const numbers = [];
for (let n = 1; n <= 9; n += 1) {
numbers.push(n);
}
const answer = [];
for (let n = 0; n <= 3; n += 1) {
const index = Math.floor(Math.random() * numbers.length);
answer.push(numbers[index]);
numbers.splice(index, 1);
}
console.log(answer);
const tries = [];
function checkInput(input) {
// 숫자 4개 입력 했는지 검사
if (input.length !== 4) {
return alert('숫자를 4개 입력해주세요');
}
// 중복 숫자 검사
if (new Set(input).size !== 4) {
return alert('중복된 숫자는 제거해서 다시 입력해주세요.');
}
// 이미 시도한 숫자 검사
if (tries.includes(input)) {
return alert('이미 시도한 값입니다. 다른 값을 입력해주세요.');
}
return true;
}
$form.addEventListener('submit', (event) => {
event.preventDefault();
const value = $input.value;
$input.value = '';
const valid = checkInput(value);
// 결과
if (!valid) return;
if (answer.join('') === value) {
$logs.textContent = '홈런!';
return;
}
if (tries.length >= 9) {
const message = document.createTextNode(
`패배! 정답은 ${answer.join('')}`
);
$logs.appendChild(message);
return;
}
// 몇 스트라이크 몇 볼인지 검사
let strike = 0;
let ball = 0;
for (let i = 0; i < answer.length; i++) {
const index = value.indexOf(answer[i]);
if (index > -1) {
if (index === i) {
strike += 1;
} else {
ball += 1;
}
}
}
// 결과 출력
$logs.append(
`${value}: ${strike} 스트라이크 ${ball} 볼`,
document.createElement('br')
);
tries.push(value);
});
</script>
</body>
</html>
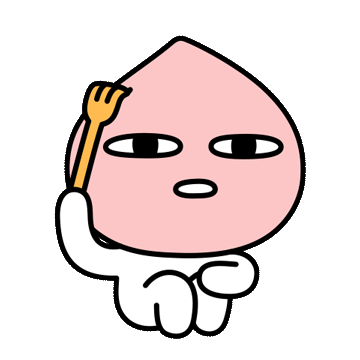
갈수록... 어렵다 .... 어려워 ...
반응형
'개발 이야기 > 개발 도서' 카테고리의 다른 글
제로초 자바스크립트 입문 <로또 추첨기> (0) | 2024.03.25 |
---|---|
제로초 자바스크립트 입문 <계산기> (0) | 2024.03.19 |
제로초 자바스크립트 입문 <끝말잇기 2> (0) | 2024.03.18 |
제로초 자바스크립트 입문 <끝말잇기 1> (0) | 2024.03.15 |
제로초 자바스크립트 입문 <window 객체> (0) | 2024.03.14 |