반응형
데이터 모킹 라이브러리
Mock Service Worker
모킹 (Mocking) 이란?Mock(모의 데이터)을 만들어서 활용하는 방식
통상적으로 data fetch를 해야하는 경우 통신을 통해 응답을 내려주는 서버가 있어야 함
서버가 없는경우
api 요청으로 내려올 데이터를 프론트에서 모킹하거나
서버의 역할을 해주는 무언가(데이터 fetch 해보기 강의에서는 github)가 필요
msw
https://mswjs.io/
Interception on the network level
Service Worker API
REST API & GraphQL support
msw | |
---|---|
Mocking | 모의 데이터 활용 |
Browser | Service worker 활용 |
REST API / GraphQL | 모두 모킹이 가능 |
설치하기
npm install msw --save-dev
yarn add msw --dev
npx msw init <PUBLIC_DIR> --save
// index.js 에 추가를 해줘야 사용가능하다.
// Start the mocking conditionally.
if (process.env.NODE_ENV === 'development') {
const { worker } = require('./mocks/browser');
worker.start();
}
import React, { useState } from 'react';
const Item = ({ name, age }) => {
return (
<li>
name: {name} / age: {age}
</li>
);
};
const url =
'https://raw.githubusercontent.com/techoi/raw-data-api/main/simple-api.json';
export default function TestMoking() {
const [data, setData] = useState(null);
const [error, setError] = useState(null);
const handleClick = () => {
fetch(url)
.then((Response) => {
return Response.json();
})
.then((json) => {
setData(json.data);
})
.catch((error) => {
setError(`Something Wrong: ${error}`);
});
};
if (error) return <p>{error}</p>;
return (
<div>
<button onClick={handleClick}>데이터 가져오기</button>
{data && (
<ul>
{data.people.map((person) => {
<Item
key={`${person.name}-${person.age}`}
name={person.name}
age={person.age}
/>;
})}
</ul>
)}
</div>
);
}
Query parameters
Response patching
Mocking error responses
Context status
msw | |
---|---|
mock | handler / browser 만 있어도 동작 |
public | npx msw init |
기타 커스텀 | query, patching, error |
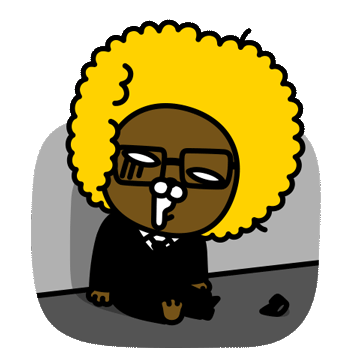
반응형
'개발 이야기 > Front-end' 카테고리의 다른 글
React 공식문서로 디테일 잡기 #7 (0) | 2023.03.26 |
---|---|
React 라이브러리 #3 (0) | 2023.03.23 |
React 공식문서로 디테일 잡기 #6 (0) | 2023.03.21 |
React 라이브러리 (0) | 2023.03.20 |
React 공식문서로 디테일 잡기 #5 (0) | 2023.03.19 |